Command-line interface
Creating and Deploying a CLI for a Python Application
Basics of CLI
CLI stands for Command Line Interface, a text-based interface used to interact with software and operating systems by typing commands into a terminal or console window. A CLI allows users to execute commands, navigate the file system, and manage system resources directly by typing commands and receiving text-based output.
Creating a CLI for a Python Application
To create a CLI for a piece of working code in Python, you can follow these conceptual steps:
Define the Functionality:
Identify the functionality you want to expose through the CLI. This includes the commands, arguments, and options your CLI will support.
Use a Library for CLI Parsing:
Python has several libraries to help with creating CLIs, such as
argparse
,click
, andtyper
. These libraries make it easier to define commands, arguments, and options.
Implement the CLI:
Write a Python script that uses the chosen library to parse command-line arguments and invoke the corresponding functions from your working code.
Example Using argparse
Define the functionality:
def add(a, b): return a + b def subtract(a, b): return a - b
Implement the CLI using
argparse
:import argparse parser = argparse.ArgumentParser(description="A simple calculator CLI") subparsers = parser.add_subparsers(dest='command') add_parser = subparsers.add_parser('add', help='Add two numbers') add_parser.add_argument('a', type=float, help='First number') add_parser.add_argument('b', type=float, help='Second number') subtract_parser = subparsers.add_parser('subtract', help='Subtract two numbers') subtract_parser.add_argument('a', type=float, help='First number') subtract_parser.add_argument('b', type=float, help='Second number') args = parser.parse_args() if args.command == 'add': result = add(args.a, args.b) print(f"The result is {result}") elif args.command == 'subtract': result = subtract(args.a, args.b) print(f"The result is {result}") else: parser.print_help()
Example Using click
Define the functionality:
def add(a, b): return a + b def subtract(a, b): return a - b
Implement the CLI using
click
:import click @click.group() def cli(): pass @cli.command() @click.argument('a', type=float) @click.argument('b', type=float) def add_command(a, b): result = add(a, b) click.echo(f"The result is {result}") @cli.command() @click.argument('a', type=float) @click.argument('b', type=float) def subtract_command(a, b): result = subtract(a, b) click.echo(f"The result is {result}") if __name__ == '__main__': cli()
Deploying the CLI in a Docker Container for Reuse on the AIMPF Platform via Seven Bridges
To make the CLI application reusable and easily deployable by all users on the AIMPF platform via Seven Bridges, we’ll wrap it in a Docker container. Docker containers provide a consistent runtime environment, ensuring that the application runs the same way on any system. We’ll then deploy this Dockerized CLI to the Seven Bridges platform, which is a cloud-based environment for tool/workflow building and analysis.
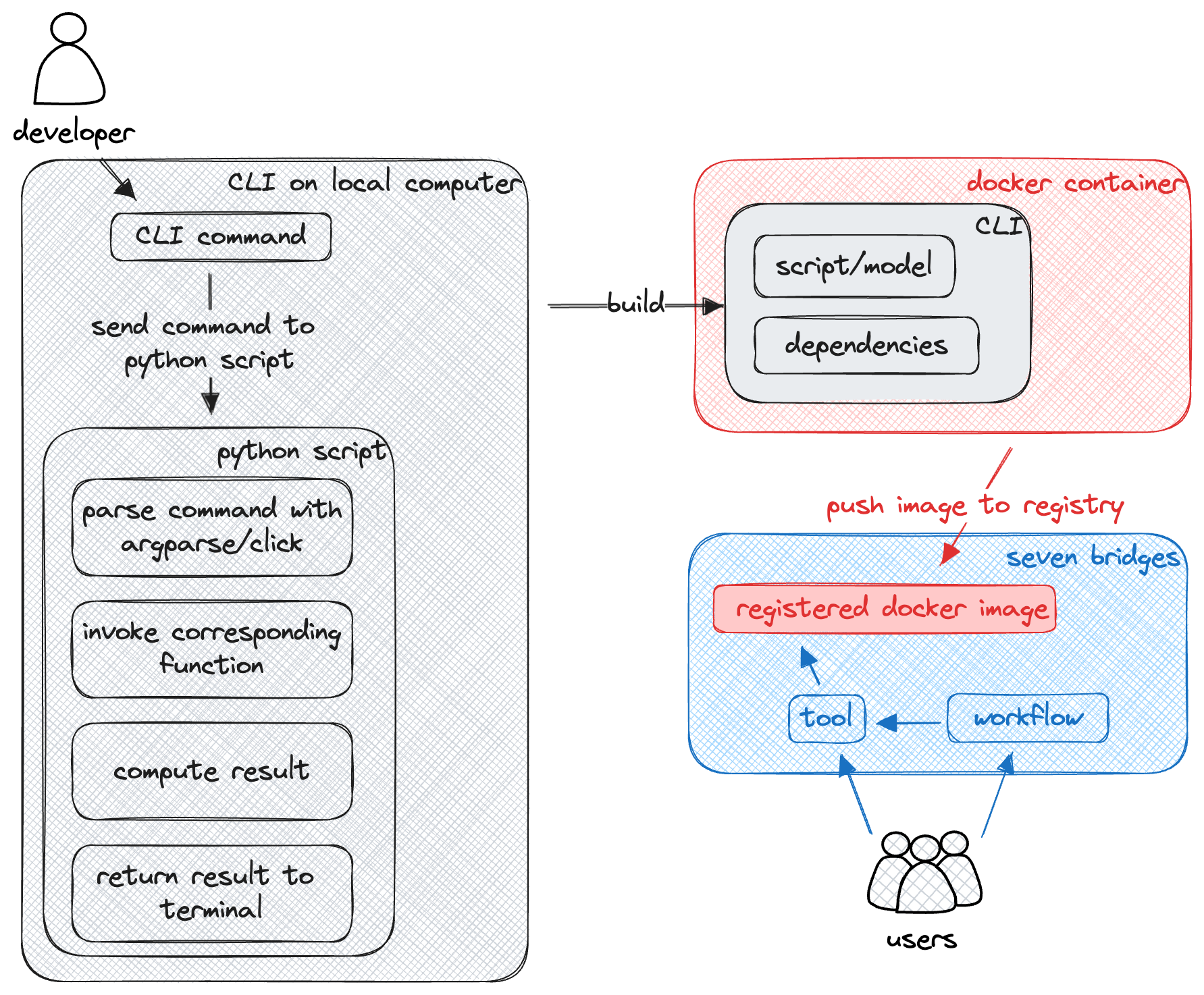
Figure 1. Schematic representation of the steps to deploy a CLI application. The diagram shows the conceptual steps involved in creating a Dockerized CLI application and deploying it on the Seven Bridges platform for reuse by all users.
Conceptual Steps to Deploy the CLI Application
To deploy the CLI application on the Seven Bridges platform, follow these steps:
Dockerize the CLI Application:
Create a Dockerfile that sets up the environment and includes the CLI application. The Dockerfile should define the base image, install necessary dependencies, and configure the application to run in a container.
Build and Test the Docker Image:
Build the Docker image from the Dockerfile and test it locally to ensure it functions correctly. This involves running the container and verifying that the CLI commands produce the expected output.
Push the Docker Image to a Container Registry:
Tag and push the Docker image to a container registry (e.g., Docker Hub, Google Container Registry) to make it accessible for deployment. This step makes the image available to be pulled and run on other systems.
Deploy on Seven Bridges:
Log in to the Seven Bridges platform and create a new task. Configure the task by specifying the Docker image URL from your container registry. Set up the input parameters for the CLI commands and run the task. Once deployed, the CLI application can be used by all users on the Seven Bridges platform.
Summary
By following these steps, we can create a reusable and easily deployable CLI application that can be run by all users on the AIMPF platform via Seven Bridges. Dockerizing the application ensures consistency, while Seven Bridges provides a powerful cloud-based environment for bioinformatics analysis. This approach simplifies deployment and allows users to focus on their analysis without worrying about the underlying infrastructure.